Using Catching Exceptions and Continuing With the Same Code Block Python
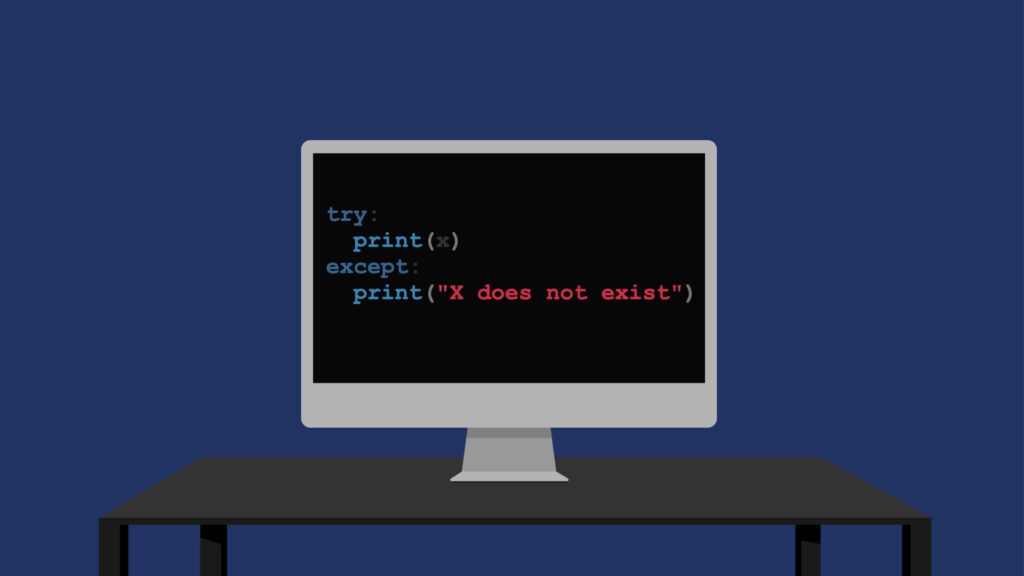
In Python, there is no such thing as try-catch.
Instead, there is a try-except structure dedicated for error handling. This works similarly to the try-catch you've seen in some other languages.
For example:
try: print(x) except: print("Exception thrown. x does not exist.")
Output:
Exception thrown. x does not exist.
- Here the program tries to run the code inside the
try
block. - If it fails, the
except
block catches the exception raised.
Try-Except Pattern in Python Error Handling
In Python, faulty expressions raise errors and exceptions that crash your program.
This is where the built-in error handling mechanism can save your day. Instead of letting your program crash, you handle the errors during runtime and the program execution continues.
The error handling pattern in Python consists of the following:
- The
try
block. It runs a piece of code. If this piece of code fails, an exception is raised. - The
except
block. This block catches the possible error raised thetry
block. - Theoptional
finally
block. This runs a piece of code regardless of whether there were errors or not. This is an optional block of code you don't need in the error handling scheme.
As an example, you can use the try-except structure to check if a name is not defined:
try: print(x) except: print("Exception thrown. x does not exist.")
In reality, this is bad practice. This is because we don't define the type of error we expect in the except
block.
To fix this, you need to know that if x
is not defined, a NameError
is raised.
Now you can expect a possible NameError
in the except block. This makes the code more understandable:
try: print(x) except NameError: print("NameError: x is not defined.")
Output:
NameError: x is not defined.
Now, what if there is more than one exception that can occur? No problem. Python lets you define as many except
blocks as needed.
Multiple Exceptions in Python Error Handling
In Python, you can have multiple except
blocks for handling different types of errors separately.
For instance, let's divide two numbers by one another. Before doing this, there are two things that can go wrong:
- One or both of the numbers are not defined.
- The denominator is zero.
The former causes a NameError
. The latter issue causes an ZeroDivisionError
. So in the try-except structure, we want to handle both exceptions separately. Thus, we need two except blocks for the different cases.
Let's turn this into code:
try: result = x / y except ZeroDivisionError: print("Make sure no divisions by 0 are made.") except NameError: print("Make sure both numbers are defined.")
As x
and y
are not defined, there will be an error message thanks to our careful error handling:
Make sure both numbers are defined
Now, let's define x
and y
, but let's make y
zero:
try: x = 10 y = 0 result = x / y except ZeroDivisionError: print("Make sure no divisions by 0 are made.") except NameError: print("Make sure both numbers are defined.")
This results in an exception of dividing by zero:
Make sure no divisions by 0 are made.
Finally, let's write the code so that it causes no errors:
try: x = 10 y = 5 result = x / y except ZeroDivisionError: print("Make sure no divisions by 0 are made.") except NameError: print("Make sure both numbers are defined.")
Now there is no error message. This means the program ran successfully.
Else Block in Python Error Handling
You can add an optional else
block after except
blocks in Python. This runs a piece of code if there were no errors thrown.
For example:
try: x = 15 y = 5 result = x / y print(result) except ZeroDivisionError: print("Make sure no divisions by 0 are made.") else: print("There were no errors.")
Finally Block in Python Error Handling
The finally
block is an optional block that runs regardless of whether there were errors or not.
For example:
try: y = 10 print(y) except: print("There was an error") else: print("There were no errors.") finally: print("Process completed.")
Output:
The number is 10 There were no errors. Process completed.
Conclusion
In Python, there is no such thing as try-catch. Instead, Python uses the try-except approach to deal with errors and exceptions.
Here is an example:
try: print(x) except: print("x is not defined") finally: print("Process finished")
Output:
x is not defined Process finished
Thanks for reading. I hope you enjoy it.
Happy coding.
Further Reading
50 Python Interview Questions with Answers
50+ Buzzwords of Web Development
willifordwhoas1964.blogspot.com
Source: https://www.codingem.com/try-catch-in-python/
0 Response to "Using Catching Exceptions and Continuing With the Same Code Block Python"
Post a Comment